Maybe it's difficult to understand what i want, so i'll try to explain:
imagine that you are holding [forward] key, for example W, so you are running forward. if you stop holding W key and press it twice, you will dodge (fast jump). I need to attach some function to pawn which executes on special [Dodge] key, for example Alt.
This will be work in following way: while holding W and running forward, if you press Alt - you will dodge in direction in which you are moving now. This must be as usual jump, but faster.
The function should check whether pawn is moving to some direction, and execute dodging in same direction. As I suppose the dodge is usual jump, so I can cast AddVelocity() on pawn. My question is there any bool variables which set to True while movement keys (W, A, S, D) are holding? Or i should save pawn location some time ago (for example 0.5 sec) and compare with current location to construct jump vector? Would this eat CPU much or not?
And how form this as mutator. Can I generate some inventory class which will be added to each pawn at PostBeginPlay() call?
Is it possible to implement dodge key for PlayerPawn?
- Voodoo Doll
- Average
- Posts: 42
- Joined: Wed Aug 03, 2011 2:27 am
- Location: Russian Federation
- Voodoo Doll
- Average
- Posts: 42
- Joined: Wed Aug 03, 2011 2:27 am
- Location: Russian Federation
Re: Is it possible to implement dodge key for PlayerPawn?
Yo, I made it ;))
maybe someone else need it
Code: Select all
class MyPickup expands Pickup;
var vector oldloc,curloc,x,y,z,w,a,s,d;
var int dir;
function postbeginplay(){
dir=0;
oldloc=location;
settimer(0.01,true);
}
function timer(){
dir=0;
if(owner==none) return;
getaxes(pawn(owner).viewrotation,x,y,z);
curloc=location;
curloc.z=0;
oldloc.z=0;
w=oldloc+x*6;
s=oldloc-x*6;
a=oldloc-y*6;
d=oldloc+y*6;
if(vsize(curloc-w)<5) dir=1;
if(vsize(curloc-s)<5) dir=2;
if(vsize(curloc-a)<5) dir=3;
if(vsize(curloc-d)<5) dir=4;
oldloc=curloc;
settimer(0.01,true);
}
exec function forcedodge(){
local playerpawn p;
p=playerpawn(owner);
if(p==none) return;
if(dir!=0) performdodge(dir,p);
}
function performdodge(int ddir,playerpawn p){
if(p.Physics!=PHYS_Walking) return;
GetAxes(p.Rotation,X,Y,Z);
if (ddir==1) p.Velocity=1.5*p.GroundSpeed*X+(p.Velocity Dot Y)*Y;
else if (ddir==2) p.Velocity=-1.5*p.GroundSpeed*X+(p.Velocity Dot Y)*Y;
else if (ddir==3) p.Velocity=-1.5*p.GroundSpeed*Y+(p.Velocity Dot X)*X;
else if (ddir==4) p.Velocity=1.5*p.GroundSpeed*Y+(p.Velocity Dot X)*X;
p.Velocity.Z=160;
p.PlayOwnedSound(p.JumpSound,SLOT_Talk,1.0,true,800,1.0);
if(ddir==1) p.PlayDodge(DODGE_Forward);
else if(ddir==2) p.PlayDodge(DODGE_Back);
else if(ddir==3) p.PlayDodge(DODGE_Left);
else if(ddir==4) p.PlayDodge(DODGE_Right);
p.SetPhysics(PHYS_Falling);
}
Re: Is it possible to implement dodge key for PlayerPawn?
So if I use the arrow keys for movement, i would substitute {left,right,up,down} in the var declaration, yes?
var vector oldloc,curloc,x,y,z,up,left,down,right
var vector oldloc,curloc,x,y,z,up,left,down,right
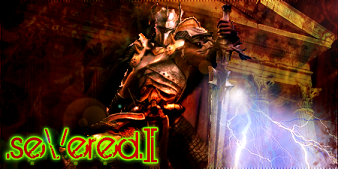
- Voodoo Doll
- Average
- Posts: 42
- Joined: Wed Aug 03, 2011 2:27 am
- Location: Russian Federation
Re: Is it possible to implement dodge key for PlayerPawn?
ChanClan
I've made it in some different way. Such implementation of this thing looks more reliable (comparing relative coordinates of pawn by timer may sometimes cause calculation errors).
It's a fragment of some inventory class script.
User is need to bind some key to forcedodge(), for example with command:
Here's an explanation how it works:
if you are running in some direction, e. g. forward, one or more your playerpawn's bWasXXXX variables become true. Once you press the key which is bound to forcedodge() call, pawn will execute dodge. It's look like press, for example, W and Alt simultaneously. I've made it since double keypressing for dodge is too twitchy, separate dodge command key is more convenient than usual dodge. The coefficients in X, Y axis (0.75*p.GroundSpeed) affects jump speed.
I had a strange issue with execution of this one time. If I'd weared some Engine.Pickup with these functions, it's not working, only in Engine.Weapon or Botpack.TournamentWeapon subclass. All working OK now.
No, w,a,s,d were my own variable names for coordinates calcs in appropriate direction. The script is independent from key bindings, since it uses location of pawn, not it's key input. Anyway, never mind.ChanClan wrote:i would substitute {left,right,up,down} in the var declaration
I've made it in some different way. Such implementation of this thing looks more reliable (comparing relative coordinates of pawn by timer may sometimes cause calculation errors).
Code: Select all
exec function forcedodge(){
local playerpawn p;
local int xdir,ydir;
local vector x,y,z;
p=playerpawn(owner);
if(p==none) return;
xdir=0;
ydir=0;
if(p.bwasforward) xdir=1;
if(p.bwasback) xdir=-1;
if(p.bwasleft) ydir=1;
if(p.bwasright) ydir=-1;
if(p.physics==phys_walking && (xdir!=0 || ydir!=0)){
getaxes(p.rotation,x,y,z);
p.velocity=(xdir*0.75*p.groundspeed+p.velocity dot x)*x+(ydir*0.75*p.groundspeed+p.velocity dot y)*y;
p.velocity.Z=160;
p.playownedsound(p.jumpsound,slot_talk,1.0,true,800,1.0);
if(xdir==0){
if(ydir==1) p.playdodge(dodge_left);
if(ydir==-1) p.playdodge(dodge_right);
}else{
if(xdir==-1) p.playdodge(dodge_back);
if(xdir==1) p.playdodge(dodge_forward);
}
p.setphysics(phys_falling);
}
}
User is need to bind some key to forcedodge(), for example with command:
Code: Select all
set input alt forcedodge
if you are running in some direction, e. g. forward, one or more your playerpawn's bWasXXXX variables become true. Once you press the key which is bound to forcedodge() call, pawn will execute dodge. It's look like press, for example, W and Alt simultaneously. I've made it since double keypressing for dodge is too twitchy, separate dodge command key is more convenient than usual dodge. The coefficients in X, Y axis (0.75*p.GroundSpeed) affects jump speed.
I had a strange issue with execution of this one time. If I'd weared some Engine.Pickup with these functions, it's not working, only in Engine.Weapon or Botpack.TournamentWeapon subclass. All working OK now.
Re: Is it possible to implement dodge key for PlayerPawn?
Fascinating... and you have no problems with ace or is it implemented on the server side?
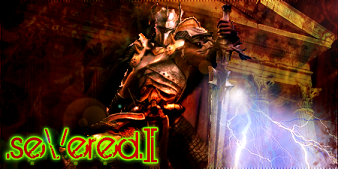
- Voodoo Doll
- Average
- Posts: 42
- Joined: Wed Aug 03, 2011 2:27 am
- Location: Russian Federation
Re: Is it possible to implement dodge key for PlayerPawn?
ChanClan, it's not tested on server yet. This thing uses same implementation as the weapon discussed in my another thread, so i think it needs replication of launching function (this one called forcedodge()) from clients to server.